原文链接: https://leetcode-cn.com/problems/maximum-difference-between-node-and-ancestor
英文原文
Given the root
of a binary tree, find the maximum value v
for which there exist different nodes a
and b
where v = |a.val - b.val|
and a
is an ancestor of b
.
A node a
is an ancestor of b
if either: any child of a
is equal to b
or any child of a
is an ancestor of b
.
Example 1:
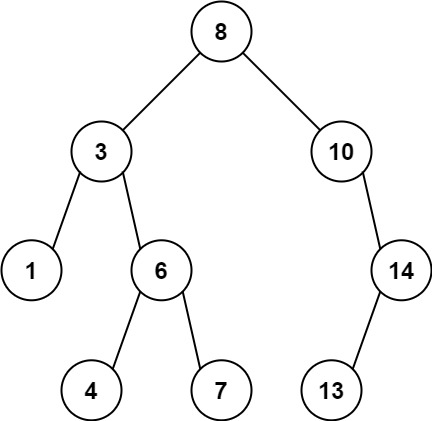
Input: root = [8,3,10,1,6,null,14,null,null,4,7,13] Output: 7 Explanation: We have various ancestor-node differences, some of which are given below : |8 - 3| = 5 |3 - 7| = 4 |8 - 1| = 7 |10 - 13| = 3 Among all possible differences, the maximum value of 7 is obtained by |8 - 1| = 7.
Example 2:
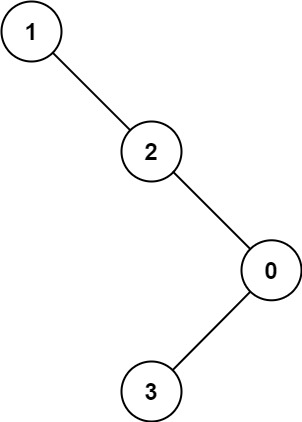
Input: root = [1,null,2,null,0,3] Output: 3
Constraints:
- The number of nodes in the tree is in the range
[2, 5000]
. 0 <= Node.val <= 105
中文题目
给定二叉树的根节点 root
,找出存在于 不同 节点 A
和 B
之间的最大值 V
,其中 V = |A.val - B.val|
,且 A
是 B
的祖先。
(如果 A 的任何子节点之一为 B,或者 A 的任何子节点是 B 的祖先,那么我们认为 A 是 B 的祖先)
示例 1:
输入:root = [8,3,10,1,6,null,14,null,null,4,7,13] 输出:7 解释: 我们有大量的节点与其祖先的差值,其中一些如下: |8 - 3| = 5 |3 - 7| = 4 |8 - 1| = 7 |10 - 13| = 3 在所有可能的差值中,最大值 7 由 |8 - 1| = 7 得出。
示例 2:
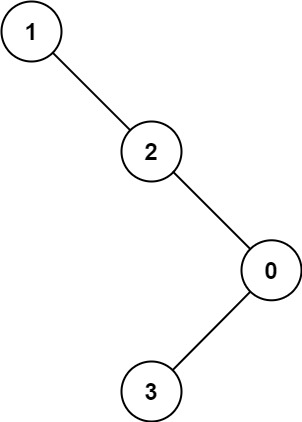
输入:root = [1,null,2,null,0,3] 输出:3
提示:
- 树中的节点数在
2
到5000
之间。 0 <= Node.val <= 105
通过代码
高赞题解
思路:
使用全局变量保存最大差值,进行dfs,每次递归查找当前路径的最大值和最小值,到达叶子节点时使用当前路径的最大值和最小值更新全局变量。
class Solution {
int res = Integer.MIN_VALUE;
public int maxAncestorDiff(TreeNode root) {
if (root == null) return 0;
//如果当前节点没有子节点,则直接返回
helper(root, root.val, root.val);
return res;
}
/**
* 每条从根节点到叶子节点的路径中的最大值和最小值,并求出差值更新全局变量
*/
private void helper(TreeNode node, int max, int min) {
if (node == null) return;
max = Math.max(node.val, max);
min = Math.min(node.val, min);
//到达叶子节点,求最大差值
if (node.left == null && node.right == null) {
res = Math.max(res, Math.abs(max - min));
}
helper(node.left, max, min);
helper(node.right, max, min);
}
}
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
10448 | 15506 | 67.4% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|