原文链接: https://leetcode-cn.com/problems/binary-search-tree-to-greater-sum-tree
英文原文
Given the root
of a Binary Search Tree (BST), convert it to a Greater Tree such that every key of the original BST is changed to the original key plus the sum of all keys greater than the original key in BST.
As a reminder, a binary search tree is a tree that satisfies these constraints:
- The left subtree of a node contains only nodes with keys less than the node's key.
- The right subtree of a node contains only nodes with keys greater than the node's key.
- Both the left and right subtrees must also be binary search trees.
Example 1:
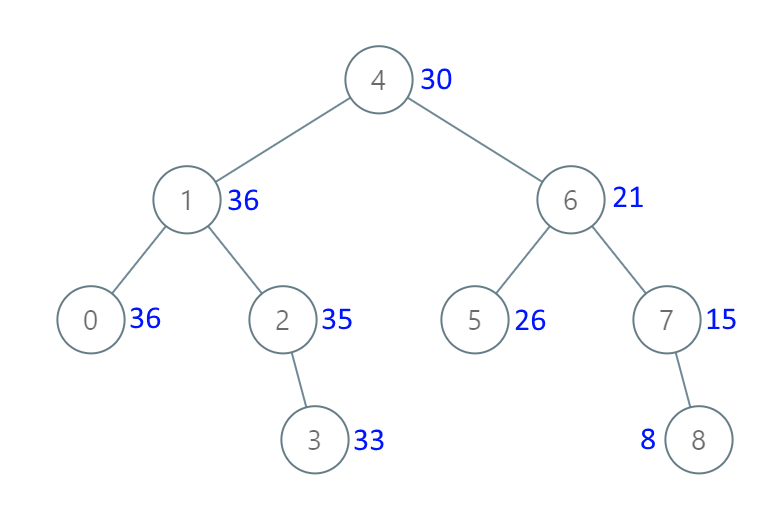
Input: root = [4,1,6,0,2,5,7,null,null,null,3,null,null,null,8] Output: [30,36,21,36,35,26,15,null,null,null,33,null,null,null,8]
Example 2:
Input: root = [0,null,1] Output: [1,null,1]
Example 3:
Input: root = [1,0,2] Output: [3,3,2]
Example 4:
Input: root = [3,2,4,1] Output: [7,9,4,10]
Constraints:
- The number of nodes in the tree is in the range
[1, 100]
. 0 <= Node.val <= 100
- All the values in the tree are unique.
root
is guaranteed to be a valid binary search tree.
Note: This question is the same as 538: https://leetcode.com/problems/convert-bst-to-greater-tree/
中文题目
给定一个二叉搜索树,请将它的每个节点的值替换成树中大于或者等于该节点值的所有节点值之和。
提醒一下,二叉搜索树满足下列约束条件:
- 节点的左子树仅包含键 小于 节点键的节点。
- 节点的右子树仅包含键 大于 节点键的节点。
- 左右子树也必须是二叉搜索树。
注意:该题目与 538: https://leetcode-cn.com/problems/convert-bst-to-greater-tree/ 相同
示例 1:
输入:[4,1,6,0,2,5,7,null,null,null,3,null,null,null,8] 输出:[30,36,21,36,35,26,15,null,null,null,33,null,null,null,8]
示例 2:
输入:root = [0,null,1] 输出:[1,null,1]
示例 3:
输入:root = [1,0,2] 输出:[3,3,2]
示例 4:
输入:root = [3,2,4,1] 输出:[7,9,4,10]
提示:
- 树中的节点数介于
1
和100
之间。 - 每个节点的值介于
0
和100
之间。 - 树中的所有值 互不相同 。
- 给定的树为二叉搜索树。
通过代码
高赞题解
解题思路
本题是关于二叉搜索树的问题,那我们第一想到的就是中序遍历,这是二叉搜索树的一个非常重要的性质,二叉搜索树的中序遍历是一个递增的有序序列。本道题我们需要将其转换为累加树,使得每个节点的值是原来的节点值加上所有大于它的节点值之和。那我们看下面的例子:
观察累加前中序遍历与累加后中序遍历,我们会发现,其实后者就是前者的一个从后的累加结果。那问题就迎刃而解了,我们只需反向中序遍历即可,并把每次的节点值进行累加,就能得到最终的累加树。而且这样保证了我们对每个节点只访问了一次。
代码
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
int sum = 0;
public TreeNode bstToGst(TreeNode root) {
if(root != null){
bstToGst(root.right);
sum = sum + root.val;
root.val = sum;
bstToGst(root.left);
}
return root;
}
}
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
26858 | 33813 | 79.4% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|