原文链接: https://leetcode-cn.com/problems/binary-tree-level-order-traversal-ii
英文原文
Given the root
of a binary tree, return the bottom-up level order traversal of its nodes' values. (i.e., from left to right, level by level from leaf to root).
Example 1:
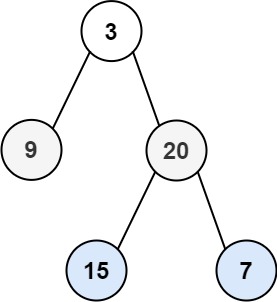
Input: root = [3,9,20,null,null,15,7] Output: [[15,7],[9,20],[3]]
Example 2:
Input: root = [1] Output: [[1]]
Example 3:
Input: root = [] Output: []
Constraints:
- The number of nodes in the tree is in the range
[0, 2000]
. -1000 <= Node.val <= 1000
中文题目
给定一个二叉树,返回其节点值自底向上的层序遍历。 (即按从叶子节点所在层到根节点所在的层,逐层从左向右遍历)
例如:
给定二叉树 [3,9,20,null,null,15,7]
,
3 / \ 9 20 / \ 15 7
返回其自底向上的层序遍历为:
[ [15,7], [9,20], [3] ]
通过代码
高赞题解
class Solution:
def levelOrderBottom(self, root):
queue = [] # 结果列表
cur = [root] # 接下来要循环的当前层节点,存的是节点
while cur: # 当前层存在结点时
cur_layer_val = [] # 初始化当前层结果列表为空,存的是val
next_layer_node = [] # 初始化下一层结点列表为空
for node in cur: # 遍历当前层的每一个结点
if node: # 如果该结点不为空,则进行记录
cur_layer_val.append(node.val) # 将该结点的值加入当前层结果列表的末尾
next_layer_node.extend([node.left, node.right]) # 将该结点的左右孩子结点加入到下一层结点列表
if cur_layer_val: # 只要当前层结果列表不为空
queue.insert(0, cur_layer_val) # 则把当前层结果列表插入到队列首端
cur = next_layer_node # 下一层的结点变成当前层,接着循环
return queue # 返回结果队列
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
173164 | 247242 | 70.0% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|
相似题目
题目 | 难度 |
---|---|
二叉树的层序遍历 | 中等 |
二叉树的层平均值 | 简单 |