原文链接: https://leetcode-cn.com/problems/delete-nodes-and-return-forest
英文原文
Given the root
of a binary tree, each node in the tree has a distinct value.
After deleting all nodes with a value in to_delete
, we are left with a forest (a disjoint union of trees).
Return the roots of the trees in the remaining forest. You may return the result in any order.
Example 1:
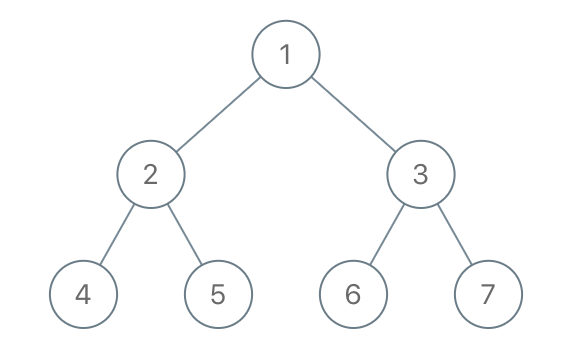
Input: root = [1,2,3,4,5,6,7], to_delete = [3,5] Output: [[1,2,null,4],[6],[7]]
Example 2:
Input: root = [1,2,4,null,3], to_delete = [3] Output: [[1,2,4]]
Constraints:
- The number of nodes in the given tree is at most
1000
. - Each node has a distinct value between
1
and1000
. to_delete.length <= 1000
to_delete
contains distinct values between1
and1000
.
中文题目
给出二叉树的根节点 root
,树上每个节点都有一个不同的值。
如果节点值在 to_delete
中出现,我们就把该节点从树上删去,最后得到一个森林(一些不相交的树构成的集合)。
返回森林中的每棵树。你可以按任意顺序组织答案。
示例 1:
输入:root = [1,2,3,4,5,6,7], to_delete = [3,5] 输出:[[1,2,null,4],[6],[7]]
示例 2:
输入:root = [1,2,4,null,3], to_delete = [3] 输出:[[1,2,4]]
提示:
- 树中的节点数最大为
1000
。 - 每个节点都有一个介于
1
到1000
之间的值,且各不相同。 to_delete.length <= 1000
to_delete
包含一些从1
到1000
、各不相同的值。
通过代码
高赞题解
解题思路
首先把to_delete变成set,此处不多说;
节点进入结果当中,有2个条件:
- 不被删除
- 父节点不存在
因此在遍历过程中,将parentExists标志传递给子节点,子递归就可以选择是否加入到结果。
另外,如果子节点被删除,父节点的left、right字段需要更新。
代码
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
Set<Integer> toDelete;
public List<TreeNode> delNodes(TreeNode root, int[] to_delete) {
toDelete = Arrays.stream(to_delete).boxed().collect(Collectors.toSet());
List<TreeNode> ans = new ArrayList<>();
helper(root, ans, false);
return ans;
}
boolean helper(TreeNode root, List<TreeNode> ans, boolean parentExists) {
boolean del = false;
if (root == null) {
return del;
}
del = toDelete.contains(root.val);
if (helper(root.left, ans, !del)) {
root.left = null;
}
if (helper(root.right, ans, !del)) {
root.right = null;
}
if (!del && !parentExists) {
ans.add(root);
}
return del;
}
}
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
11992 | 19023 | 63.0% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|