原文链接: https://leetcode-cn.com/problems/longest-zigzag-path-in-a-binary-tree
英文原文
You are given the root
of a binary tree.
A ZigZag path for a binary tree is defined as follow:
- Choose any node in the binary tree and a direction (right or left).
- If the current direction is right, move to the right child of the current node; otherwise, move to the left child.
- Change the direction from right to left or from left to right.
- Repeat the second and third steps until you can't move in the tree.
Zigzag length is defined as the number of nodes visited - 1. (A single node has a length of 0).
Return the longest ZigZag path contained in that tree.
Example 1:
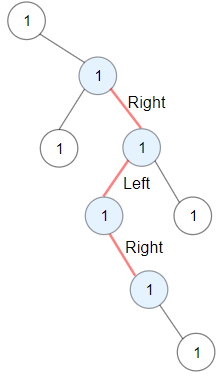
Input: root = [1,null,1,1,1,null,null,1,1,null,1,null,null,null,1,null,1] Output: 3 Explanation: Longest ZigZag path in blue nodes (right -> left -> right).
Example 2:
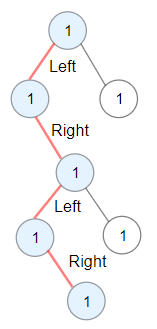
Input: root = [1,1,1,null,1,null,null,1,1,null,1] Output: 4 Explanation: Longest ZigZag path in blue nodes (left -> right -> left -> right).
Example 3:
Input: root = [1] Output: 0
Constraints:
- The number of nodes in the tree is in the range
[1, 5 * 104]
. 1 <= Node.val <= 100
中文题目
给你一棵以 root
为根的二叉树,二叉树中的交错路径定义如下:
- 选择二叉树中 任意 节点和一个方向(左或者右)。
- 如果前进方向为右,那么移动到当前节点的的右子节点,否则移动到它的左子节点。
- 改变前进方向:左变右或者右变左。
- 重复第二步和第三步,直到你在树中无法继续移动。
交错路径的长度定义为:访问过的节点数目 - 1(单个节点的路径长度为 0 )。
请你返回给定树中最长 交错路径 的长度。
示例 1:
输入:root = [1,null,1,1,1,null,null,1,1,null,1,null,null,null,1,null,1] 输出:3 解释:蓝色节点为树中最长交错路径(右 -> 左 -> 右)。
示例 2:
输入:root = [1,1,1,null,1,null,null,1,1,null,1] 输出:4 解释:蓝色节点为树中最长交错路径(左 -> 右 -> 左 -> 右)。
示例 3:
输入:root = [1] 输出:0
提示:
- 每棵树最多有
50000
个节点。 - 每个节点的值在
[1, 100]
之间。
通过代码
高赞题解
思路:
记录当前结点是左/右孩子,即记录当前路径的方向
搜索其孩子时,根据上一条路径方向判断
如果当前路径方向相反,路径长度+1,如果相同,路径长度置为1
class Solution {
int ans=0;
void dfs(TreeNode* root,int dir,int dis){//(当前结点,左/右孩子,路径长度)
if(!root)return;//空结点返回
ans=max(ans,dis);//更新最大值
if(dir){//如果当前结点是其父结点的右孩子
dfs(root->left,0,dis+1);//搜索其左孩子时,满足ZigZig,路径长度+1
dfs(root->right,1,1);//搜索其右孩子时,不满足ZigZig,路径长度置为1
}
else{//如果当前结点是其父结点的左孩子
dfs(root->left,0,1);//搜索其左孩子时,不满足ZigZig,路径长度置为1
dfs(root->right,1,dis+1);//搜索其右孩子时,满足ZigZig,路径长度+1
}
}
public:
int longestZigZag(TreeNode* root) {
dfs(root->left,0,1);//0左节点
dfs(root->right,1,1);//1右结点
return ans;
}
};
3月9日 updata
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
8593 | 16648 | 51.6% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|