原文链接: https://leetcode-cn.com/problems/merge-in-between-linked-lists
英文原文
You are given two linked lists: list1
and list2
of sizes n
and m
respectively.
Remove list1
's nodes from the ath
node to the bth
node, and put list2
in their place.
The blue edges and nodes in the following figure indicate the result:
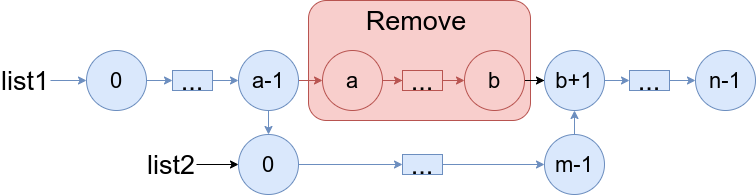
Build the result list and return its head.
Example 1:
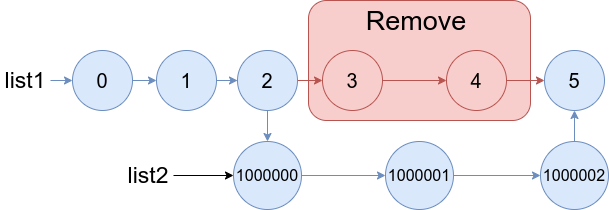
Input: list1 = [0,1,2,3,4,5], a = 3, b = 4, list2 = [1000000,1000001,1000002] Output: [0,1,2,1000000,1000001,1000002,5] Explanation: We remove the nodes 3 and 4 and put the entire list2 in their place. The blue edges and nodes in the above figure indicate the result.
Example 2:
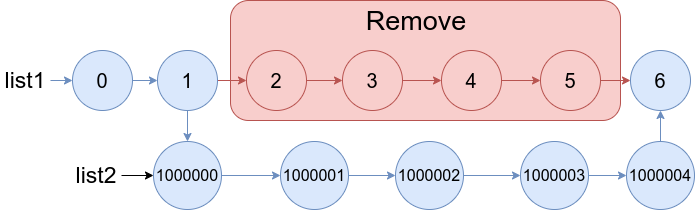
Input: list1 = [0,1,2,3,4,5,6], a = 2, b = 5, list2 = [1000000,1000001,1000002,1000003,1000004] Output: [0,1,1000000,1000001,1000002,1000003,1000004,6] Explanation: The blue edges and nodes in the above figure indicate the result.
Constraints:
3 <= list1.length <= 104
1 <= a <= b < list1.length - 1
1 <= list2.length <= 104
中文题目
给你两个链表 list1
和 list2
,它们包含的元素分别为 n
个和 m
个。
请你将 list1
中下标从 a
到 b
的全部节点都删除,并将list2
接在被删除节点的位置。
下图中蓝色边和节点展示了操作后的结果:
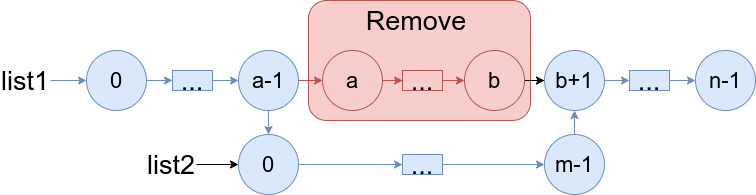
请你返回结果链表的头指针。
示例 1:
输入:list1 = [0,1,2,3,4,5], a = 3, b = 4, list2 = [1000000,1000001,1000002] 输出:[0,1,2,1000000,1000001,1000002,5] 解释:我们删除 list1 中下标为 3 和 4 的两个节点,并将 list2 接在该位置。上图中蓝色的边和节点为答案链表。
示例 2:
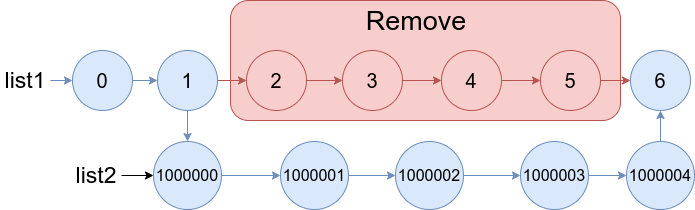
输入:list1 = [0,1,2,3,4,5,6], a = 2, b = 5, list2 = [1000000,1000001,1000002,1000003,1000004] 输出:[0,1,1000000,1000001,1000002,1000003,1000004,6] 解释:上图中蓝色的边和节点为答案链表。
提示:
3 <= list1.length <= 104
1 <= a <= b < list1.length - 1
1 <= list2.length <= 104
通过代码
高赞题解
解题思路
此处撰写解题思路
代码
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode mergeInBetween(ListNode list1, int a, int b, ListNode list2) {
ListNode l1=list1;
ListNode l2=list2;
ListNode tmp=new ListNode(0);
int i=0,j=0,z=0;
while(l1.next != null){
if(i == a-1){
tmp=l1.next;
l1.next=l2;
break;
}else{
l1=l1.next;
i++;
}
}
while(tmp != null){
if(i+j == b){
break;
}else{
tmp=tmp.next;
j++;
}
}
ListNode l11=list1;
while(l11 != null){
l11=l11.next;
if(l11.next == null){
l11.next=tmp;
break;
}
}
return list1;
}
}
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
13806 | 18240 | 75.7% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|