原文链接: https://leetcode-cn.com/problems/remove-nth-node-from-end-of-list
英文原文
Given the head
of a linked list, remove the nth
node from the end of the list and return its head.
Example 1:
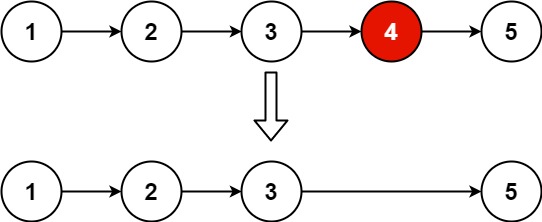
Input: head = [1,2,3,4,5], n = 2 Output: [1,2,3,5]
Example 2:
Input: head = [1], n = 1 Output: []
Example 3:
Input: head = [1,2], n = 1 Output: [1]
Constraints:
- The number of nodes in the list is
sz
. 1 <= sz <= 30
0 <= Node.val <= 100
1 <= n <= sz
Follow up: Could you do this in one pass?
中文题目
给你一个链表,删除链表的倒数第 n
个结点,并且返回链表的头结点。
示例 1:
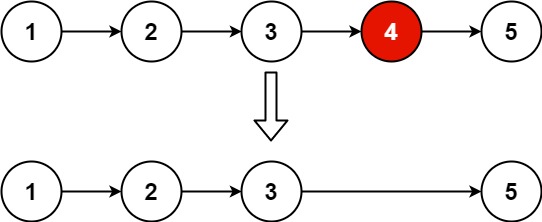
输入:head = [1,2,3,4,5], n = 2 输出:[1,2,3,5]
示例 2:
输入:head = [1], n = 1 输出:[]
示例 3:
输入:head = [1,2], n = 1 输出:[1]
提示:
- 链表中结点的数目为
sz
1 <= sz <= 30
0 <= Node.val <= 100
1 <= n <= sz
进阶:你能尝试使用一趟扫描实现吗?
通过代码
高赞题解
题目解析
采取双重遍历肯定是可以解决问题的,但题目要求我们一次遍历解决问题,那我们的思路得发散一下。
我们可以设想假设设定了双指针 p
和 q
的话,当 q
指向末尾的 NULL
,p
与 q
之间相隔的元素个数为 n
时,那么删除掉 p
的下一个指针就完成了要求。
- 设置虚拟节点
dummyHead
指向head
- 设定双指针
p
和q
,初始都指向虚拟节点dummyHead
- 移动
q
,直到p
与q
之间相隔的元素个数为n
- 同时移动
p
与q
,直到q
指向的为NULL
- 将
p
的下一个节点指向下下个节点
动画描述
{:width=”400”}
{:align=”center”}
代码实现
[]class Solution { public: ListNode* removeNthFromEnd(ListNode* head, int n) { ListNode* dummyHead = new ListNode(0); dummyHead->next = head; ListNode* p = dummyHead; ListNode* q = dummyHead; for( int i = 0 ; i < n + 1 ; i ++ ){ q = q->next; } while(q){ p = p->next; q = q->next; } ListNode* delNode = p->next; p->next = delNode->next; delete delNode; ListNode* retNode = dummyHead->next; delete dummyHead; return retNode; } };
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
578191 | 1339458 | 43.2% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|