原文链接: https://leetcode-cn.com/problems/check-if-move-is-legal
英文原文
You are given a 0-indexed 8 x 8
grid board
, where board[r][c]
represents the cell (r, c)
on a game board. On the board, free cells are represented by '.'
, white cells are represented by 'W'
, and black cells are represented by 'B'
.
Each move in this game consists of choosing a free cell and changing it to the color you are playing as (either white or black). However, a move is only legal if, after changing it, the cell becomes the endpoint of a good line (horizontal, vertical, or diagonal).
A good line is a line of three or more cells (including the endpoints) where the endpoints of the line are one color, and the remaining cells in the middle are the opposite color (no cells in the line are free). You can find examples for good lines in the figure below:
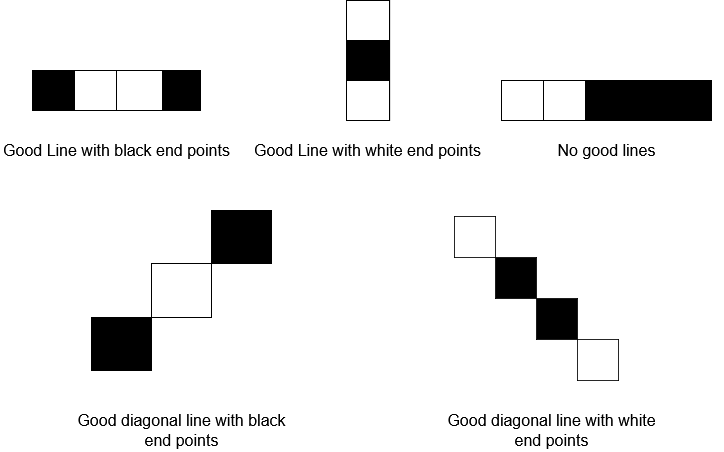
Given two integers rMove
and cMove
and a character color
representing the color you are playing as (white or black), return true
if changing cell (rMove, cMove)
to color color
is a legal move, or false
if it is not legal.
Example 1:
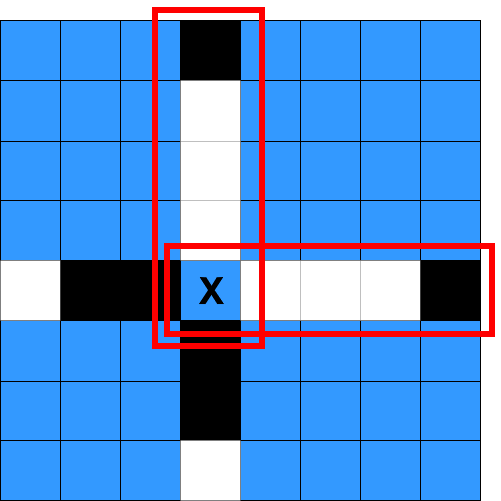
Input: board = [[".",".",".","B",".",".",".","."],[".",".",".","W",".",".",".","."],[".",".",".","W",".",".",".","."],[".",".",".","W",".",".",".","."],["W","B","B",".","W","W","W","B"],[".",".",".","B",".",".",".","."],[".",".",".","B",".",".",".","."],[".",".",".","W",".",".",".","."]], rMove = 4, cMove = 3, color = "B" Output: true Explanation: '.', 'W', and 'B' are represented by the colors blue, white, and black respectively, and cell (rMove, cMove) is marked with an 'X'. The two good lines with the chosen cell as an endpoint are annotated above with the red rectangles.
Example 2:
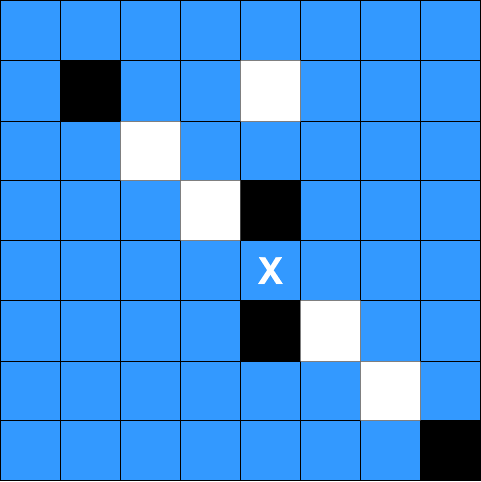
Input: board = [[".",".",".",".",".",".",".","."],[".","B",".",".","W",".",".","."],[".",".","W",".",".",".",".","."],[".",".",".","W","B",".",".","."],[".",".",".",".",".",".",".","."],[".",".",".",".","B","W",".","."],[".",".",".",".",".",".","W","."],[".",".",".",".",".",".",".","B"]], rMove = 4, cMove = 4, color = "W" Output: false Explanation: While there are good lines with the chosen cell as a middle cell, there are no good lines with the chosen cell as an endpoint.
Constraints:
board.length == board[r].length == 8
0 <= rMove, cMove < 8
board[rMove][cMove] == '.'
color
is either'B'
or'W'
.
中文题目
给你一个下标从 0 开始的 8 x 8
网格 board
,其中 board[r][c]
表示游戏棋盘上的格子 (r, c)
。棋盘上空格用 '.'
表示,白色格子用 'W'
表示,黑色格子用 'B'
表示。
游戏中每次操作步骤为:选择一个空格子,将它变成你正在执行的颜色(要么白色,要么黑色)。但是,合法 操作必须满足:涂色后这个格子是 好线段的一个端点 (好线段可以是水平的,竖直的或者是对角线)。
好线段 指的是一个包含 三个或者更多格子(包含端点格子)的线段,线段两个端点格子为 同一种颜色 ,且中间剩余格子的颜色都为 另一种颜色 (线段上不能有任何空格子)。你可以在下图找到好线段的例子:
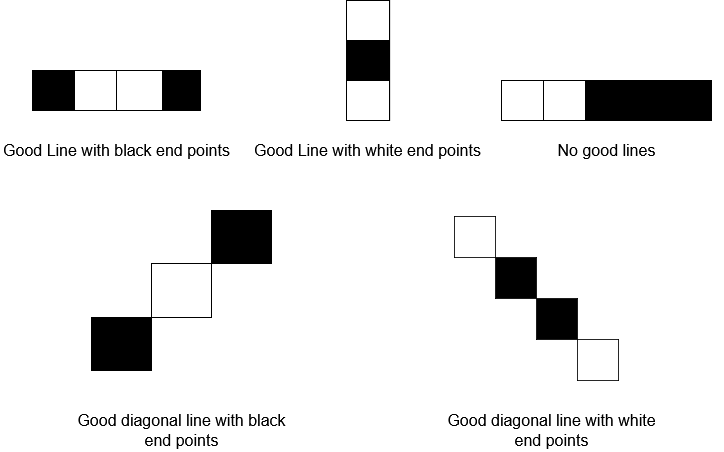
给你两个整数 rMove
和 cMove
以及一个字符 color
,表示你正在执行操作的颜色(白或者黑),如果将格子 (rMove, cMove)
变成颜色 color
后,是一个 合法 操作,那么返回 true
,如果不是合法操作返回 false
。
示例 1:
输入:board = [[".",".",".","B",".",".",".","."],[".",".",".","W",".",".",".","."],[".",".",".","W",".",".",".","."],[".",".",".","W",".",".",".","."],["W","B","B",".","W","W","W","B"],[".",".",".","B",".",".",".","."],[".",".",".","B",".",".",".","."],[".",".",".","W",".",".",".","."]], rMove = 4, cMove = 3, color = "B" 输出:true 解释:'.','W' 和 'B' 分别用颜色蓝色,白色和黑色表示。格子 (rMove, cMove) 用 'X' 标记。 以选中格子为端点的两个好线段在上图中用红色矩形标注出来了。
示例 2:
输入:board = [[".",".",".",".",".",".",".","."],[".","B",".",".","W",".",".","."],[".",".","W",".",".",".",".","."],[".",".",".","W","B",".",".","."],[".",".",".",".",".",".",".","."],[".",".",".",".","B","W",".","."],[".",".",".",".",".",".","W","."],[".",".",".",".",".",".",".","B"]], rMove = 4, cMove = 4, color = "W" 输出:false 解释:虽然选中格子涂色后,棋盘上产生了好线段,但选中格子是作为中间格子,没有产生以选中格子为端点的好线段。
提示:
board.length == board[r].length == 8
0 <= rMove, cMove < 8
board[rMove][cMove] == '.'
color
要么是'B'
要么是'W'
。
通过代码
高赞题解
5827. 检查操作是否合法
第二道题。
给了咱一个二维数组和一个点的坐标,问改了这个点能不能以这个点为端点生成新的线段。
模拟题,只要把八个方向都遍历一遍就好了。
class Solution {
public:
bool checkMove(vector<vector<char>>& board, int rMove, int cMove, char color) {
int n = board.size();
int m = board[0].size();
bool flag = false;
for(int i = cMove + 1; i < m; ++i){ //向右侧遍历
if(board[rMove][i] == '.') break; //遇到空白,退出
if(board[rMove][i] != color) flag = true; //遇到不同的颜色,记录下来
else if(flag) return true; //遇到另一个端点且中间有不同的颜色
else break; //遇到相同的颜色,但中间没有不同的颜色,退出
}
flag = false;
for(int i = cMove - 1; i >= 0; --i){ //向左侧遍历
if(board[rMove][i] == '.') break;
if(board[rMove][i] != color) flag = true;
else if(flag) return true;
else break;
}
flag = false;
for(int i = rMove + 1; i < n; ++i){ //向下遍历
if(board[i][cMove] == '.') break;
if(board[i][cMove] != color) flag = true;
else if(flag) return true;
else break;
}
flag = false;
for(int i = rMove - 1; i >= 0; --i){ //向上遍历
if(board[i][cMove] == '.') break;
if(board[i][cMove] != color) flag = true;
else if(flag) return true;
else break;
}
flag = false;
for(int i = rMove + 1, j = cMove + 1; i < n && j < m; ++i, ++j){ //向右下方遍历
if(board[i][j] == '.') break;
if(board[i][j] != color) flag = true;
else if(flag) return true;
else break;
}
flag = false;
for(int i = rMove - 1, j = cMove - 1; i >= 0 && j >= 0; --i, --j){ //向左上方遍历
if(board[i][j] == '.') break;
if(board[i][j] != color) flag = true;
else if(flag) return true;
else break;
}
flag = false;
for(int i = rMove - 1, j = cMove + 1; i >= 0 && j < m; --i, ++j){ //向右上方遍历
if(board[i][j] == '.') break;
if(board[i][j] != color) flag = true;
else if(flag) return true;
else break;
}
flag = false;
for(int i = rMove + 1, j = cMove - 1; i < n && j >= 0; ++i, --j){ //向左下方遍历
if(board[i][j] == '.') break;
if(board[i][j] != color) flag = true;
else if(flag) return true;
else break;
}
return false;
}
};
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
2595 | 6089 | 42.6% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|