原文链接: https://leetcode-cn.com/problems/binary-tree-right-side-view
英文原文
Given the root
of a binary tree, imagine yourself standing on the right side of it, return the values of the nodes you can see ordered from top to bottom.
Example 1:
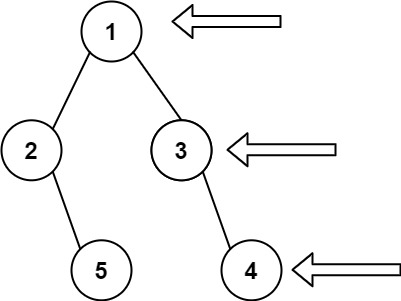
Input: root = [1,2,3,null,5,null,4] Output: [1,3,4]
Example 2:
Input: root = [1,null,3] Output: [1,3]
Example 3:
Input: root = [] Output: []
Constraints:
- The number of nodes in the tree is in the range
[0, 100]
. -100 <= Node.val <= 100
中文题目
给定一个二叉树的 根节点 root
,想象自己站在它的右侧,按照从顶部到底部的顺序,返回从右侧所能看到的节点值。
示例 1:
输入: [1,2,3,null,5,null,4] 输出: [1,3,4]
示例 2:
输入: [1,null,3] 输出: [1,3]
示例 3:
输入: [] 输出: []
提示:
- 二叉树的节点个数的范围是
[0,100]
-100 <= Node.val <= 100
通过代码
高赞题解
🙋今日打卡
今天的「官方题解」里的「视频部分」是我录的嗷,走过路过别错喂 ✪ω✪, 任何建议欢迎大家抛出,谢谢!
本文补充一下视频讲解的文字版本 ✪ω✪
一、BFS
思路: 利用 BFS 进行层次遍历,记录下每层的最后一个元素。
时间复杂度: $O(N)$,每个节点都入队出队了 1 次。
空间复杂度: $O(N)$,使用了额外的队列空间。
class Solution {
public List<Integer> rightSideView(TreeNode root) {
List<Integer> res = new ArrayList<>();
if (root == null) {
return res;
}
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
while (!queue.isEmpty()) {
int size = queue.size();
for (int i = 0; i < size; i++) {
TreeNode node = queue.poll();
if (node.left != null) {
queue.offer(node.left);
}
if (node.right != null) {
queue.offer(node.right);
}
if (i == size - 1) { //将当前层的最后一个节点放入结果列表
res.add(node.val);
}
}
}
return res;
}
}
二、DFS (时间100%)
思路: 我们按照 「根结点 -> 右子树 -> 左子树」 的顺序访问,就可以保证每层都是最先访问最右边的节点的。
(与先序遍历 「根结点 -> 左子树 -> 右子树」 正好相反,先序遍历每层最先访问的是最左边的节点)
时间复杂度: $O(N)$,每个节点都访问了 1 次。
空间复杂度: $O(N)$,因为这不是一棵平衡二叉树,二叉树的深度最少是 $logN$, 最坏的情况下会退化成一条链表,深度就是 $N$,因此递归时使用的栈空间是 $O(N)$ 的。
class Solution {
List<Integer> res = new ArrayList<>();
public List<Integer> rightSideView(TreeNode root) {
dfs(root, 0); // 从根节点开始访问,根节点深度是0
return res;
}
private void dfs(TreeNode root, int depth) {
if (root == null) {
return;
}
// 先访问 当前节点,再递归地访问 右子树 和 左子树。
if (depth == res.size()) { // 如果当前节点所在深度还没有出现在res里,说明在该深度下当前节点是第一个被访问的节点,因此将当前节点加入res中。
res.add(root.val);
}
depth++;
dfs(root.right, depth);
dfs(root.left, depth);
}
}
❤️ 谢谢观看,麻烦赏个「爱心赞」再走吧~
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
154235 | 236392 | 65.2% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|
相似题目
题目 | 难度 |
---|---|
填充每个节点的下一个右侧节点指针 | 中等 |
二叉树的边界 | 中等 |