原文链接: https://leetcode-cn.com/problems/count-nodes-with-the-highest-score
英文原文
There is a binary tree rooted at 0
consisting of n
nodes. The nodes are labeled from 0
to n - 1
. You are given a 0-indexed integer array parents
representing the tree, where parents[i]
is the parent of node i
. Since node 0
is the root, parents[0] == -1
.
Each node has a score. To find the score of a node, consider if the node and the edges connected to it were removed. The tree would become one or more non-empty subtrees. The size of a subtree is the number of the nodes in it. The score of the node is the product of the sizes of all those subtrees.
Return the number of nodes that have the highest score.
Example 1:
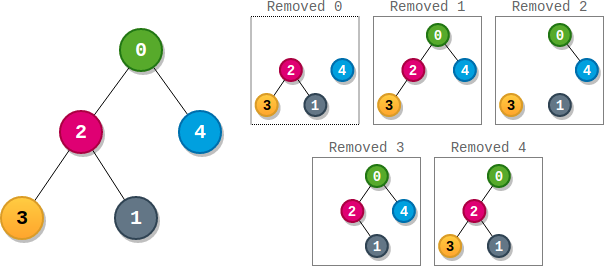
Input: parents = [-1,2,0,2,0] Output: 3 Explanation: - The score of node 0 is: 3 * 1 = 3 - The score of node 1 is: 4 = 4 - The score of node 2 is: 1 * 1 * 2 = 2 - The score of node 3 is: 4 = 4 - The score of node 4 is: 4 = 4 The highest score is 4, and three nodes (node 1, node 3, and node 4) have the highest score.
Example 2:
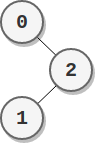
Input: parents = [-1,2,0] Output: 2 Explanation: - The score of node 0 is: 2 = 2 - The score of node 1 is: 2 = 2 - The score of node 2 is: 1 * 1 = 1 The highest score is 2, and two nodes (node 0 and node 1) have the highest score.
Constraints:
n == parents.length
2 <= n <= 105
parents[0] == -1
0 <= parents[i] <= n - 1
fori != 0
parents
represents a valid binary tree.
中文题目
给你一棵根节点为 0
的 二叉树 ,它总共有 n
个节点,节点编号为 0
到 n - 1
。同时给你一个下标从 0 开始的整数数组 parents
表示这棵树,其中 parents[i]
是节点 i
的父节点。由于节点 0
是根,所以 parents[0] == -1
。
一个子树的 大小 为这个子树内节点的数目。每个节点都有一个与之关联的 分数 。求出某个节点分数的方法是,将这个节点和与它相连的边全部 删除 ,剩余部分是若干个 非空 子树,这个节点的 分数 为所有这些子树 大小的乘积 。
请你返回有 最高得分 节点的 数目 。
示例 1:
输入:parents = [-1,2,0,2,0] 输出:3 解释: - 节点 0 的分数为:3 * 1 = 3 - 节点 1 的分数为:4 = 4 - 节点 2 的分数为:1 * 1 * 2 = 2 - 节点 3 的分数为:4 = 4 - 节点 4 的分数为:4 = 4 最高得分为 4 ,有三个节点得分为 4 (分别是节点 1,3 和 4 )。
示例 2:
输入:parents = [-1,2,0] 输出:2 解释: - 节点 0 的分数为:2 = 2 - 节点 1 的分数为:2 = 2 - 节点 2 的分数为:1 * 1 = 1 最高分数为 2 ,有两个节点分数为 2 (分别为节点 0 和 1 )。
提示:
n == parents.length
2 <= n <= 105
parents[0] == -1
- 对于
i != 0
,有0 <= parents[i] <= n - 1
parents
表示一棵二叉树。
通过代码
高赞题解
1.使用Map存储当前节点,以及它的子节点。
2.利用DFS查询并存储当前节点下的节点个数。
3.遍历计算各个节点删除后的得分情况(0号节点没有父节点)。
class Solution {
public int countHighestScoreNodes(int[] parents) {
Map<Integer, List<Integer>> map = new HashMap();
int[] count = new int[parents.length];
for(int i = 1; i < parents.length; i++){
List<Integer> list = new ArrayList(map.getOrDefault( parents[i], new ArrayList()));
list.add(i);
map.put(parents[i], list);
}
DFS(0, map, count);
long scoreMax = Integer.MIN_VALUE;
int nodes = 0;
for(int i = 0; i < parents.length; i++){
long score = 1;
if(parents[i] == -1){
List<Integer> list = new ArrayList( map.getOrDefault( i, new ArrayList() ) );
for(int num : list)
score *= count[num];
}else{
score = count[0] - count[i];
List<Integer> list = new ArrayList( map.getOrDefault( i, new ArrayList() ) );
for(int num : list)
score *= count[num];
}
if(scoreMax < score){
scoreMax = score;
nodes = 1;
}else if(score == scoreMax) ++nodes;
}
return nodes;
}
public void DFS(int index, Map<Integer, List<Integer>> map, int[] count){
List<Integer> list = new ArrayList( map.getOrDefault( index, new ArrayList() ) );
if(list.size() == 0){
count[index] = 1;
return;
}
for(int num : list){
DFS(num, map, count);
count[index] += count[num];
}
++count[index];
}
}
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
2765 | 7044 | 39.3% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|