原文链接: https://leetcode-cn.com/problems/reverse-nodes-in-even-length-groups
英文原文
You are given the head
of a linked list.
The nodes in the linked list are sequentially assigned to non-empty groups whose lengths form the sequence of the natural numbers (1, 2, 3, 4, ...
). The length of a group is the number of nodes assigned to it. In other words,
- The
1st
node is assigned to the first group. - The
2nd
and the3rd
nodes are assigned to the second group. - The
4th
,5th
, and6th
nodes are assigned to the third group, and so on.
Note that the length of the last group may be less than or equal to 1 + the length of the second to last group
.
Reverse the nodes in each group with an even length, and return the head
of the modified linked list.
Example 1:

Input: head = [5,2,6,3,9,1,7,3,8,4] Output: [5,6,2,3,9,1,4,8,3,7] Explanation: - The length of the first group is 1, which is odd, hence no reversal occurrs. - The length of the second group is 2, which is even, hence the nodes are reversed. - The length of the third group is 3, which is odd, hence no reversal occurrs. - The length of the last group is 4, which is even, hence the nodes are reversed.
Example 2:
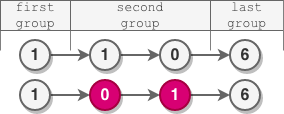
Input: head = [1,1,0,6] Output: [1,0,1,6] Explanation: - The length of the first group is 1. No reversal occurrs. - The length of the second group is 2. The nodes are reversed. - The length of the last group is 1. No reversal occurrs.
Example 3:
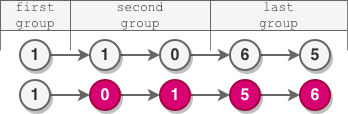
Input: head = [1,1,0,6,5] Output: [1,0,1,5,6] Explanation: - The length of the first group is 1. No reversal occurrs. - The length of the second group is 2. The nodes are reversed. - The length of the last group is 2. The nodes are reversed.
Example 4:
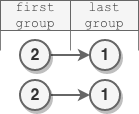
Input: head = [2,1] Output: [2,1] Explanation: - The length of the first group is 1. No reversal occurrs. - The length of the last group is 1. No reversal occurrs.
Example 5:
Input: head = [8] Output: [8] Explanation: There is only one group whose length is 1. No reversal occurrs.
Constraints:
- The number of nodes in the list is in the range
[1, 105]
. 0 <= Node.val <= 105
中文题目
给你一个链表的头节点 head
。
链表中的节点 按顺序 划分成若干 非空 组,这些非空组的长度构成一个自然数序列(1, 2, 3, 4, ...
)。一个组的 长度 就是组中分配到的节点数目。换句话说:
- 节点
1
分配给第一组 - 节点
2
和3
分配给第二组 - 节点
4
、5
和6
分配给第三组,以此类推
注意,最后一组的长度可能小于或者等于 1 + 倒数第二组的长度
。
反转 每个 偶数 长度组中的节点,并返回修改后链表的头节点 head
。
示例 1:
输入:head = [5,2,6,3,9,1,7,3,8,4] 输出:[5,6,2,3,9,1,4,8,3,7] 解释: - 第一组长度为 1 ,奇数,没有发生反转。 - 第二组长度为 2 ,偶数,节点反转。 - 第三组长度为 3 ,奇数,没有发生反转。 - 最后一组长度为 4 ,偶数,节点反转。
示例 2:
输入:head = [1,1,0,6] 输出:[1,0,1,6] 解释: - 第一组长度为 1 ,没有发生反转。 - 第二组长度为 2 ,节点反转。 - 最后一组长度为 1 ,没有发生反转。
示例 3:
输入:head = [2,1] 输出:[2,1] 解释: - 第一组长度为 1 ,没有发生反转。 - 最后一组长度为 1 ,没有发生反转。
示例 4:
输入:head = [8] 输出:[8] 解释:只有一个长度为 1 的组,没有发生反转。
提示:
- 链表中节点数目范围是
[1, 105]
0 <= Node.val <= 105
通过代码
高赞题解
func reverseEvenLengthGroups(head *ListNode) *ListNode {
var nodes []*ListNode
for node, size := head, 1; node != nil; node = node.Next {
nodes = append(nodes, node)
if len(nodes) == size || node.Next == nil { // 统计到 size 个节点,或到达链表末尾
if n := len(nodes); n%2 == 0 { // 有偶数个节点
for i := 0; i < n/2; i++ {
nodes[i].Val, nodes[n-1-i].Val = nodes[n-1-i].Val, nodes[i].Val // 直接交换元素值
}
}
nodes = nil
size++
}
}
return head
}
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
3730 | 9156 | 40.7% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|