原文链接: https://leetcode-cn.com/problems/count-complete-tree-nodes
英文原文
Given the root
of a complete binary tree, return the number of the nodes in the tree.
According to Wikipedia, every level, except possibly the last, is completely filled in a complete binary tree, and all nodes in the last level are as far left as possible. It can have between 1
and 2h
nodes inclusive at the last level h
.
Design an algorithm that runs in less than O(n)
time complexity.
Example 1:
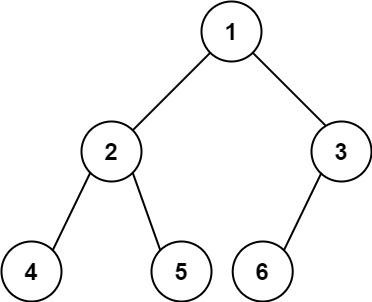
Input: root = [1,2,3,4,5,6] Output: 6
Example 2:
Input: root = [] Output: 0
Example 3:
Input: root = [1] Output: 1
Constraints:
- The number of nodes in the tree is in the range
[0, 5 * 104]
. 0 <= Node.val <= 5 * 104
- The tree is guaranteed to be complete.
中文题目
给你一棵 完全二叉树 的根节点 root
,求出该树的节点个数。
完全二叉树 的定义如下:在完全二叉树中,除了最底层节点可能没填满外,其余每层节点数都达到最大值,并且最下面一层的节点都集中在该层最左边的若干位置。若最底层为第 h
层,则该层包含 1~ 2h
个节点。
示例 1:
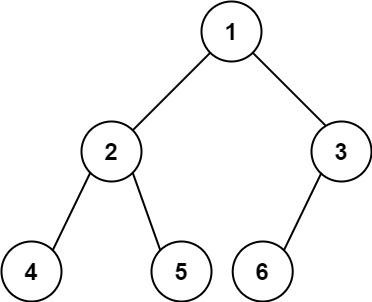
输入:root = [1,2,3,4,5,6] 输出:6
示例 2:
输入:root = [] 输出:0
示例 3:
输入:root = [1] 输出:1
提示:
- 树中节点的数目范围是
[0, 5 * 104]
0 <= Node.val <= 5 * 104
- 题目数据保证输入的树是 完全二叉树
进阶:遍历树来统计节点是一种时间复杂度为 O(n)
的简单解决方案。你可以设计一个更快的算法吗?
通过代码
高赞题解
解题思路:
对于没有约束的二叉树而言,可以很简单地想到使用下面这个递归的解法:
[]public int countNodes(TreeNode root) { if (root == null){ return 0; } return countNodes(root.left) + countNodes(root.right) + 1; }
但这是一个普适的解法,对于此题给的完全二叉树的特点没有利用起来,进一步考虑如何使用完全二叉树的特点更快解出此题。
首先需要明确完全二叉树的定义:它是一棵空树或者它的叶子节点只出在最后两层,若最后一层不满则叶子节点只在最左侧。
再来回顾一下满二叉的节点个数怎么计算,如果满二叉树的层数为h,则总节点数为:2^h - 1.
那么我们来对 root 节点的左右子树进行高度统计,分别记为 left 和 right,有以下两种结果:
left == right
。这说明,左子树一定是满二叉树,因为节点已经填充到右子树了,左子树必定已经填满了。所以左子树的节点总数我们可以直接得到,是2^left - 1
,加上当前这个 root 节点,则正好是2^left
。再对右子树进行递归统计。left != right
。说明此时最后一层不满,但倒数第二层已经满了,可以直接得到右子树的节点个数。同理,右子树节点+root
节点,总数为2^right
。再对左子树进行递归查找。
关于如何计算二叉树的层数,可以利用下面的递归来算,当然对于完全二叉树,可以利用其特点,不用递归直接算,具体请参考最后的完整代码。
[]private int countLevel(TreeNode root){ if(root == null){ return 0; } return Math.max(countLevel(root.left),countLevel(root.right)) + 1; }
如何计算 2^left
,最快的方法是移位计算,因为运算符的优先级问题,记得加括号哦。
完整版代码:
[]/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */ class Solution { public int countNodes(TreeNode root) { if(root == null){ return 0; } int left = countLevel(root.left); int right = countLevel(root.right); if(left == right){ return countNodes(root.right) + (1<<left); }else{ return countNodes(root.left) + (1<<right); } } private int countLevel(TreeNode root){ int level = 0; while(root != null){ level++; root = root.left; } return level; } }
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
128336 | 163830 | 78.3% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|
相似题目
题目 | 难度 |
---|---|
最接近的二叉搜索树值 | 简单 |