原文链接: https://leetcode-cn.com/problems/longest-univalue-path
英文原文
Given the root
of a binary tree, return the length of the longest path, where each node in the path has the same value. This path may or may not pass through the root.
The length of the path between two nodes is represented by the number of edges between them.
Example 1:
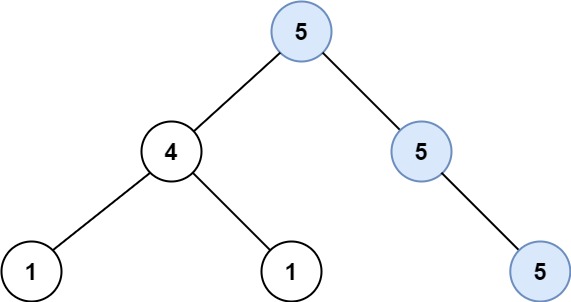
Input: root = [5,4,5,1,1,5] Output: 2
Example 2:
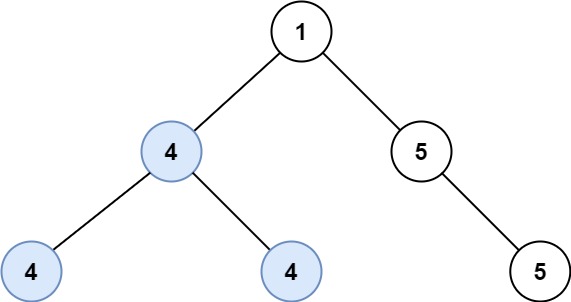
Input: root = [1,4,5,4,4,5] Output: 2
Constraints:
- The number of nodes in the tree is in the range
[0, 104]
. -1000 <= Node.val <= 1000
- The depth of the tree will not exceed
1000
.
中文题目
给定一个二叉树,找到最长的路径,这个路径中的每个节点具有相同值。 这条路径可以经过也可以不经过根节点。
注意:两个节点之间的路径长度由它们之间的边数表示。
示例 1:
输入:
5 / \ 4 5 / \ \ 1 1 5
输出:
2
示例 2:
输入:
1 / \ 4 5 / \ \ 4 4 5
输出:
2
注意: 给定的二叉树不超过10000个结点。 树的高度不超过1000。
通过代码
官方题解
方法:递归
思路
我们可以将任何路径(具有相同值的节点)看作是最多两个从其根延伸出的箭头。
具体地说,路径的根将是唯一节点,因此该节点的父节点不会出现在该路径中,而箭头将是根在该路径中只有一个子节点的路径。
然后,对于每个节点,我们想知道向左延伸的最长箭头和向右延伸的最长箭头是什么?我们可以用递归来解决这个问题。
算法
令 arrow_length(node)
为从节点 node
延伸出的最长箭头的长度。如果 node.Left
存在且与节点 node
具有相同的值,则该值就会是 1 + arrow_length(node.left)
。在 node.right
存在的情况下也是一样。
当我们计算箭头长度时,候选答案将是该节点在两个方向上的箭头之和。我们将这些候选答案记录下来,并返回最佳答案。
[9VRybv6i-Java]class Solution { int ans; public int longestUnivaluePath(TreeNode root) { ans = 0; arrowLength(root); return ans; } public int arrowLength(TreeNode node) { if (node == null) return 0; int left = arrowLength(node.left); int right = arrowLength(node.right); int arrowLeft = 0, arrowRight = 0; if (node.left != null && node.left.val == node.val) { arrowLeft += left + 1; } if (node.right != null && node.right.val == node.val) { arrowRight += right + 1; } ans = Math.max(ans, arrowLeft + arrowRight); return Math.max(arrowLeft, arrowRight); } }
[9VRybv6i-Python]class Solution(object): def longestUnivaluePath(self, root): self.ans = 0 def arrow_length(node): if not node: return 0 left_length = arrow_length(node.left) right_length = arrow_length(node.right) left_arrow = right_arrow = 0 if node.left and node.left.val == node.val: left_arrow = left_length + 1 if node.right and node.right.val == node.val: right_arrow = right_length + 1 self.ans = max(self.ans, left_arrow + right_arrow) return max(left_arrow, right_arrow) arrow_length(root) return self.ans
复杂度分析
时间复杂度:$O(N)$,其中 $N$ 是树中节点数。我们处理每个节点一次。
空间复杂度:$O(H)$,其中 $H$ 是树的高度。我们的递归调用栈可以达到 $H$ 层的深度。
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
39723 | 89667 | 44.3% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|
相似题目
题目 | 难度 |
---|---|
二叉树中的最大路径和 | 困难 |
统计同值子树 | 中等 |
路径总和 III | 中等 |