原文链接: https://leetcode-cn.com/problems/all-paths-from-source-to-target
英文原文
Given a directed acyclic graph (DAG) of n
nodes labeled from 0
to n - 1
, find all possible paths from node 0
to node n - 1
and return them in any order.
The graph is given as follows: graph[i]
is a list of all nodes you can visit from node i
(i.e., there is a directed edge from node i
to node graph[i][j]
).
Example 1:
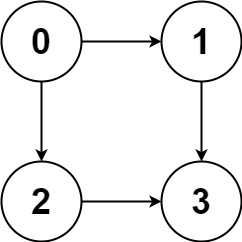
Input: graph = [[1,2],[3],[3],[]] Output: [[0,1,3],[0,2,3]] Explanation: There are two paths: 0 -> 1 -> 3 and 0 -> 2 -> 3.
Example 2:
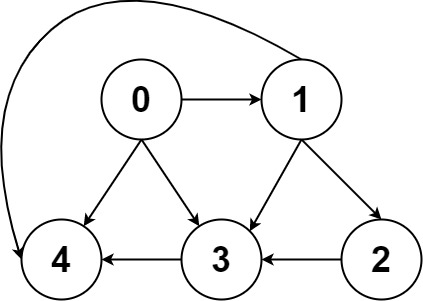
Input: graph = [[4,3,1],[3,2,4],[3],[4],[]] Output: [[0,4],[0,3,4],[0,1,3,4],[0,1,2,3,4],[0,1,4]]
Example 3:
Input: graph = [[1],[]] Output: [[0,1]]
Example 4:
Input: graph = [[1,2,3],[2],[3],[]] Output: [[0,1,2,3],[0,2,3],[0,3]]
Example 5:
Input: graph = [[1,3],[2],[3],[]] Output: [[0,1,2,3],[0,3]]
Constraints:
n == graph.length
2 <= n <= 15
0 <= graph[i][j] < n
graph[i][j] != i
(i.e., there will be no self-loops).- All the elements of
graph[i]
are unique. - The input graph is guaranteed to be a DAG.
中文题目
给你一个有 n
个节点的 有向无环图(DAG),请你找出所有从节点 0
到节点 n-1
的路径并输出(不要求按特定顺序)
二维数组的第 i
个数组中的单元都表示有向图中 i
号节点所能到达的下一些节点,空就是没有下一个结点了。
译者注:有向图是有方向的,即规定了 a→b 你就不能从 b→a 。
示例 1:
输入:graph = [[1,2],[3],[3],[]] 输出:[[0,1,3],[0,2,3]] 解释:有两条路径 0 -> 1 -> 3 和 0 -> 2 -> 3
示例 2:
输入:graph = [[4,3,1],[3,2,4],[3],[4],[]] 输出:[[0,4],[0,3,4],[0,1,3,4],[0,1,2,3,4],[0,1,4]]
示例 3:
输入:graph = [[1],[]] 输出:[[0,1]]
示例 4:
输入:graph = [[1,2,3],[2],[3],[]] 输出:[[0,1,2,3],[0,2,3],[0,3]]
示例 5:
输入:graph = [[1,3],[2],[3],[]] 输出:[[0,1,2,3],[0,3]]
提示:
n == graph.length
2 <= n <= 15
0 <= graph[i][j] < n
graph[i][j] != i
(即,不存在自环)graph[i]
中的所有元素 互不相同- 保证输入为 有向无环图(DAG)
通过代码
高赞题解
DFS
$n$ 只有 $15$,且要求输出所有方案,因此最直观的解决方案是使用 DFS
进行爆搜。
起始将 $0$ 进行加入当前答案,当 $n - 1$ 被添加到当前答案时,说明找到了一条从 $0$ 到 $n - 1$ 的路径,将当前答案加入结果集。
当我们决策到第 $x$ 位(非零)时,该位置所能放入的数值由第 $x - 1$ 位已经填入的数所决定,同时由于给定的 $graph$ 为有向无环图(拓扑图),因此按照第 $x - 1$ 位置的值去决策第 $x$ 位的内容,必然不会决策到已经在当前答案的数值,否则会与 $graph$ 为有向无环图(拓扑图)的先决条件冲突。
换句话说,与一般的爆搜不同的是,我们不再需要 $vis$ 数组来记录某个点是否已经在当前答案中。
代码:
[]class Solution { int[][] g; int n; List<List<Integer>> ans = new ArrayList<>(); List<Integer> cur = new ArrayList<>(); public List<List<Integer>> allPathsSourceTarget(int[][] graph) { g = graph; n = g.length; cur.add(0); dfs(0); return ans; } void dfs(int u) { if (u == n - 1) { ans.add(new ArrayList<>(cur)); return ; } for (int next : g[u]) { cur.add(next); dfs(next); cur.remove(cur.size() - 1); } } }
- 时间复杂度:共有 $n$ 个节点,每个节点有选和不选两种决策,总的方案数最多为 $2^n$,对于每个方案最坏情况需要 $O(n)$ 的复杂度进行拷贝并添加到结果集。整体复杂度为 $O(n * 2^n)$
- 空间复杂度:最多有 $2^n$ 种方案,每个方案最多有 $n$ 个元素。整体复杂度为 $O(n * 2^n)$
最后
如果有帮助到你,请给题解点个赞和收藏,让更多的人看到 ~ (“▔□▔)/
也欢迎你 关注我(公主号后台回复「送书」即可参与看题解学算法送实体书长期活动)或 加入「组队打卡」小群 ,提供写「证明」&「思路」的高质量题解。
所有题解已经加入 刷题指南,欢迎 star 哦 ~
统计信息
通过次数 | 提交次数 | AC比率 |
---|---|---|
47665 | 60279 | 79.1% |
提交历史
提交时间 | 提交结果 | 执行时间 | 内存消耗 | 语言 |
---|